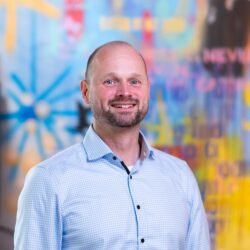
Do you want to know how to get your error logs to appear in Google Error Reporting? In this blog, I will show you how to configure your application’s logging so that errors logged in Google Cloud Logging will automatically appear in Google Error Reporting: By configuring structured logging and adding two properties to the log record, this integration can be done quickly.
Google Error Reporting
Google Error Reporting is a nice service that aggregates errors detected in your applications and allows for quick response and resolution.
Here is why I find it invaluable:
- It provides quick insights into various error types.
- It supports notifications for new errors across different channels.
- It allows direct linking to tickets in incident management systems.
Notifications can be sent to various channels, from SMS and Slack to the Google Cloud App . In short, it provides out-of-the-box support for incident response management.
Error logs do not appear in Error Reporting
The logs of all our applications running in Google Cloud are already captured and written to Google Cloud Logging. Whenever there is an Exception in the log, it is automatically detected by Google Error Reporting. But there is one snag: errors that are logged by the application without throwing an exception, do not appear in error reporting.
Reported error event log
According to the documentation, errors can be reported through the Google Error Reporting API or by writing a ReportedErrorEvent record in the log. Calling the API directly isn’t ideal because it duplicates the error in the log. Instead, I discovered that adding serviceContext
and reportLocation
to the log record allows Error Reporting to recognize it.
Delving into the Google Cloud Logging Python library, I found that the Google Cloud Logging handler will react to the json_fields
attribute on the log record, merging these fields into the structured log output automatically.
Add the error context as json fields
So now you know what to: Just add the required JSON fields to the log record, as shown in this code snippet:
def addErrorContextFilter(record: logging.LogRecord) ->bool:
"""
adds the attribute `json_fields` to the LogRecords of severity ERROR
to ensure that the log is detected by Google Error Reporting.
"""
if record.levelno >= logging.ERROR:
setattr(
record,
"json_fields",
{
"serviceContext": {"service": "demo", "version": "1.0"},
"context": {
"reportLocation": {
"filePath": record.pathname,
"lineNumber": record.lineno,
"functionName": record.funcName,
}
},
},
)
return True
Of course, you should specify your application’s service name and version in a slightly more intelligent manner. Now all you have to do is add the filter to the standard logger.
Setup Python Logging for use Google Logging
The following code snippet demonstrates how to set up structured logging in Python and add the error context filter.
import google.cloud.logging_v2
import logging
def main():
##Setup the Python error logging to use Google Cloud Logging
credentials, project = gcloud_config_helper.default()
client = google.cloud.logging_v2.Client(
project=project,
credentials=credentials)
client.setup_logging(log_level=logging.INFO)
## Now add the ErrorContext filter
logging.getLogger().addFilter(addErrorContextFilter)
Conclusion
With just a few lines of code, you can make any error log appear in Google Error Reporting. Ideally, Google could add this to the standard library by default, simplifying the process even further.
Image by
Michael Geiger from
Pixabay